Bugfender SDK for jQuery
Bugfender is a game-changing platform that logs every detail your users experience and feeds the data directly to an easy-to-use web console. Bugfender SDK is multi-platform and available for mobile and web apps, so you can use the same tool for all your apps.
Specifically for jQuery developers, Bugfender offers powerful capabilities to remotely monitor and debug any log or error. You can easily reproduce and fix issues using our detailed log viewer and the stack trace of exceptions.
If you don't have a Bugfender account yet, go to Bugfender Signup and come back here.
Install
In order to get started using the Bugfender JavaScript SDK, you will need to load the Bugfender script to the top of your application, before all other scripts. Alternatively, you can also install the SDK via a package manager.
To install Bugfender, add the following script
tag before your app script
tag:
<script defer src="https://js.bugfender.com/bugfender-v2.js"></script>
<script defer src="your-script.js"></script>
Note that to respect the loading order the script tags must have the defer attribute and not async. If async is used, the order is not guaranteed so there might be logs & errors from your app that Bugfender won't catch.
Configure
Configuring the Bugfender SDK for your JavaScript application is super easy! You just need to initialize the SDK and do it as early as possible in your application's lifecycle.
In the your-script.js
file or your html
, initialize Bugfender:
Bugfender.init({
appKey: '<YOUR_APP_KEY_HERE>',
// apiURL: 'https://api.bugfender.com/', //Usually not required, should be used for on-premises or custom data centers
// baseURL: 'https://dashboard.bugfender.com', //Usually not required, should be used for on-premises or custom data centers
// overrideConsoleMethods: true,
// printToConsole: true,
// registerErrorHandler: true,
// logBrowserEvents: true,
// logUIEvents: true,
// version: '',
// build: '',
});
Alternatively, it can also be initialized inline:
<script type="module">
Bugfender.init({
appKey: '<YOUR_APP_KEY_HERE>',
// apiURL: 'https://api.bugfender.com',
// baseURL: 'https://dashboard.bugfender.com',
// overrideConsoleMethods: true,
// printToConsole: true,
// registerErrorHandler: true,
// logBrowserEvents: true,
// logUIEvents: true,
// version: '',
// build: '',
});
</script>
Note that type="module"
is necessary in conjunction with defer to ensure correct loading order.
Remember to change YOUR_APP_KEY_HERE with the app key of your app. You can get an app key by signing up to Bugfender.
For all the available options see our reference.
Usage
Send logs
After the successful installation and configuration, you can now use our APIs in your JavaScript files.
Bugfender.log('This is a log');
Similarly, the SDK provides other methods to send logs to the server:
Bugfender.trace()
Bugfender.info()
Bugfender.log()
Bugfender.warn()
Bugfender.error()
Bugfender.fatal()
The signature of these methods is the same as the window.console
equivalents.
Bugfender also accepts string substitutions like the console
object does:
for (var i=0; i<5; i++) {
Bugfender.log("Hello, %s. You've called me %d times.", "Bob", i+1);
}
For more information, check out the MDN docs on console.
Capturing console
logs
Probably you might want to get all you app logs in your log viewer, to do this, when you init Bugfender you can enable the console override. For this there’s the overrideConsoleMethods
option you can enable/enable. By default the SDK will capture window.console calls.
Device associated data
Once your application is running on several devices, it will be useful to know which device belongs to whom. You can associate information to a device as if it were a dictionary:
Bugfender.setDeviceKey('key', 'value');
Bugfender.removeDeviceKey('key');
This will later on let you search this device by “key” in the Bugfender Dashboard and you can use any of the associated data to filter our your device list.
Send issues
Bugfender allows you to send issues to the server. An issue is similar to an exception but they are showed in the issues section and you can send issues any time from the app, even if the device is not enabled in the system. Issues are useful to keep track of important errors that you can detect in your code.
Bugfender.sendIssue('Issue title', 'Description of the issue');
Issues are displayed in a separate section of the Dashboard. When you send an issue, all logs in cache for the current session are displayed in the Dashboard, even if the device is not enabled for sending logs.
Collect user feedback
Getting feedback from the final users is one of the most important things for an app developer. Good user feedback allows you to detect errors in your app and helps you to understand better your product.
Sending User Feedback automatically sends all cached logs for the current session, even if the device is not enabled for sending logs.
Built-in feedback modal
You can send user feedback with our ready-made UI like this:
Bugfender.getUserFeedback().then((result) => {
if (result.isSent) {
// User sent the feedback
// `result.feedbackURL` contains the Bugfender feedback URL
} else {
// User closed the modal without sending the feedback
}
});
And you will get something like this:
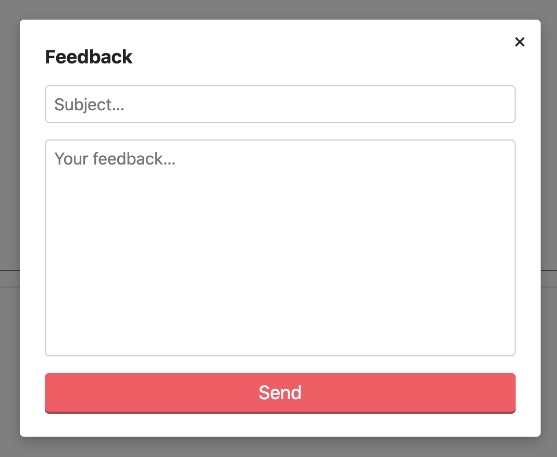
This method accepts an options object which allows to customise the modal strings. We also provide CSS variables to customise the styling (see below).
--bf-backdrop-bg: rgba(0, 0, 0, .5);
--bf-border-radius: 4px;
--bf-color: #222;
--bf-font-heading: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, Helvetica, Arial, sans-serif, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol";
--bf-font: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, Helvetica, Arial, sans-serif, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol";
--bf-input-border: 1px solid #ccc;
--bf-modal-shadow: 0px 4px 12px 0px rgba(0, 0, 0, 0.25);
--bf-placeholder-color: #757575;
--bf-submit-bg: #ff5060;
--bf-submit-color: #fff;
--bf-submit-shadow: 0 2px 0 0 #a93e48;
--bf-z-index: 9999;
Send feedback using your UI
You can send user feedback directly if you got the feedback with your own view controller. To send it, you should do like this:
Bugfender.sendUserFeedback('Love the App!', 'You are doing a great job with it.')
Advanced Usage
Send logs with tags
Bugfender has a more advanced logging system than console.log()
which allows you to add tags, specify the file name, line name or function name.
For example, adding a tag will allow you find and filter logs easily in the Bugfender log viewer.
Bugfender.sendLog({ tag: 'tag1', text: 'this is my log' });
Please note in this case the function is sendLog()
and the passed parameter is an ILogEntry
. All of the fields in the object are optional, if you do not fill them, they will be automatically populated by Bugfender.
Using LogLevel enum
The way you access the LogLevel
enum depends on how you're consuming the Bugfender SDK.
NPM package, ES6 import
:
import { LogLevel } from '@bugfender/sdk';
NPM package, CommonJS require
:
const LogLevel = require('@bugfender/sdk').LogLevel;
Browser <script>
tag:
Bugfender.LogLevel
Disable UI events logging
Bugfender logs some UI events by default. This includes: clicks, field focus/blur, field keypresses with the resulting value (except for password
fields) and form submissions. To disable this behaviour set logUIEvents
option to false
on Bugfender initialization.
Ignore keypress value for sensitive fields
Bugfender already ignores password fields from keypress logging, but if you need to ignore a sensitive field you can use the data-bf-ignore-keypress
attribute. Note that this can be applied to a single field or a group of fields. If you want to disable this behavior for the whole page add the attribute to the html
or body
element.
<!-- Ignore a single field -->
<input placeholder="Sensitive field…" data-bf-ignore-keypress>
<!-- Ignore a group of fields. Note that all children of `form` are ignored. -->
<form data-bf-ignore-keypress>
<input placeholder="Superhero name">
<input placeholder="Superhero HQ address">
</form>
<!-- Ignore on all the fields -->
<body data-bf-ignore-keypress>
...
</body>
Note: that what's being ignored is the value of the field. The keypress event will still be registered.
Send a Crash manually
You can also manually send crash information. Usually you do not want to use this, enable automated crash reporting instead using the registerErrorHandler
option. This will force all logs in the current session to be sent, even if log sending is disabled.
Bugfender.sendCrash('Crash Title', `Ops, the app crashed, better check what's wrong.`);
API Reference
For a complete API Reference you can visit: https://js.bugfender.com.
Content Security Policy
If you have CSP enabled you'll need to grant access to the following resources:
api.bugfender.com
: for the Bugfender API.js.bugfender.com
: for the browser<script>
.
Here you have the security policy you might use to grant access to both the API & the JS library:
Content-Security-Policy: default-src api.bugfender.com js.bugfender.com;
For more information on how to configure CSP please refer to the MDN documentation.